React useState Hook
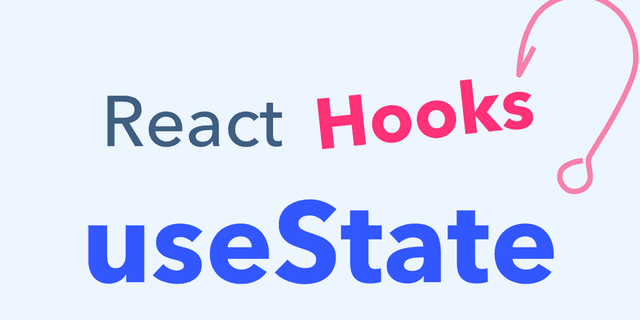
React "useState" Hook
Introduction
We can perform state-ful logic inside React functional components using the useState Hook. We can store the state of a component using the useState Hook. The state is stored by React in the javascript memory. Upon updating the state using the useState Hook, the React component function is re-executed leading to the latest state being rendered on the UI. Let us look at this in detail.
Syntax
Importing the useState Hook
The useState Hook can be imported as a named import from the 'react' library.
import { useState } from "react";
Declaring the useState Hook
The useState Hook always needs to be declared at the top-level of a component function.
- The useState Hook can be declared by executing the useState function.
- The function takes in an argument - the initial state.
- The function returns an array of 2 constants which could be retrieved by array de-structuring: the current state and a setter function that can used to set a value to the state.
const [count, setCount] = useState(0);
Detailed Working
Upon declaring a variable or a state using the useState Hook, React stores and maintains the state in the JavaScript memory. To set a value to the variable, use the setter function and pass in the new value. The setter function indicates to React that the component function needs to be re-executed, which then executes the useState function. The re-execution of the state Hook ensures the state variable gets updated with the latest value since every execution of the state Hook returns the latest value. As the state updates, it gets reflected on the DOM courtesy of React's virtual DOM. Once the component function is re-executed, the updated value gets reflected on the DOM. The execution of the state Hook in a component would not affect any other component except the component where the useState for this variable is initialized or used. Also, when the component function is reexecuted, it would not reset the variable's value to its initial value since React keeps track of the first initialization of useState Hook. React also keeps track of the current or the latest state between renders and always provides us with the most current state. So, during every subsequent execution of the state Hook and except the first execution, the initial value is ignored.
Depiction using a working example
Consider the below React component.
import React, { useState } from "react"; const App: React.FC = () => { const [count, setCount] = useState(0); const updateCountHandler = () => { setCount(count + 1); }; console.log("Executing component function"); return ( <> <h1>The counter value is: {count}</h1> <button onClick={updateCountHandler}>Increment Count</button> </> ); }; export default App;
In the above example, we declare a state count using the useState Hook. The Hook takes in the initial value of the count as the argument. As depicted, the initial state of the count is 0.
The initial UI rendered on the screen is as below.
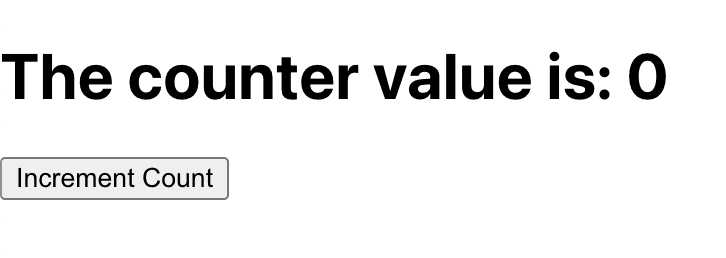
As seen in the image, the initial state of the count is rendered as 0.
In the above code snippet, notice the following 2 points:
-
Upon clicking of the button, we increment the state count by calling the setter function retrieved from the useState Hook. The setter function setCount takes in an argument and that argument is the value that we need to update the count to.
-
We have a console log in the component function to indicate when the component function is executed.
Now, let us click on the button and notice that the UI is re-rendered with an updated count value as below.
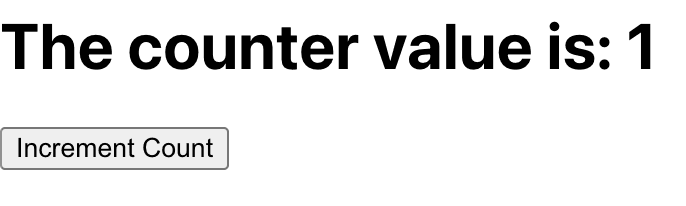
Also, notice the console log is printed everytime we update the state using the useState Hook. As explained earlier, this is due to the component function being executed/re-executed by React whenever the state is updated using the setter function. Upon executing/re-executing the component function, the useState function is executed as well and the state gets updated with the latest value. This makes sure the latest state gets rendered on the UI instantaneously.